Enough Git to Be Dangerous
Today’s blog post will cover git basics. Git is a version control system, which lets you easily store and manage historical versions of your codebase (no more emailing codeUpdate_Final_10.22.2021_actualfinal.zip
back and forth with your coworkers…)
What does git have to do with security?
- You can use git to clone (download) useful security tools
Knowing git can help you make the most of/.git/
directory findings - And you can also look for mistakenly shared tokens, keys, and so on within git-related services like GitHub.
How to use git
To be honest, you could spend years learning git
. There are lots of edge cases or weird use-cases you might find yourself in. If that’s the case, Google + Stack Overflow should be able to help you out.
What I want to cover in this section is enough git to get by (ha, ha). As is the case with a lot of systems, 20% of the commands can cover ~80% of what you need, and that’s what we’ll cover here.
git clone <git repository URL>
: use git to clone (download) a copy of the repository to your computer, including the commit history.git init
: turns a directory into a git repository. You only need to do this once, and then the rest of the git commands in this list will work.git status
: shows you the state of a git repository, and can be executed from anywhere in the git repository including sub folders. This will show which files are changed, which are committed, and which are untracked.- Changed: files that have been modified since the last commit
- Committed: files that have been staged and are ready to be pushed to the remote server.
- Untracked: files that are specifically not tracked, usually done by making a .gitignore file that says which directories or file types to ignore.
git diff:
shows you the line-by-line changes within tracked files.git add <file or directory name>
: saves a snapshot (of sorts) of the given file or directory. This is the first step in the process of pushing your code to a remote server so that others can see your changes.git commit
: once a file has been added, you can commit it, which groups it with other committed files. This is usually done with an accompanying message that gives you (and other developers) insight into why the changes were done. An example might begit commit -m "updated all print calls to use Python 3 syntax"
git push
: if you have committed a batch of files, this only updates the version history on your local machine. To share it with the remote server you need to dogit push
, and enter authentication if prompted.git push
is how you share your updates with anyone else who is using the repository.git pull
: if you are working with a team and someone else has added, committed, and pushed their changes, how do you get the latest commit from them? By doing the corollary ofgit push
, which isgit pull
.
If you find a /.git/ directory
Let’s say you’re using a web scanner and it returns a HTTP 200 status for sitename.com/.git/
Double-checking the directory
You can manually confirm that the /.git/
directory’s contents are accessible by checking these files:
/.git/config
/.git/HEAD
/.git/logs/HEAD
Your scanner might already check for these, but if not, navigating to those endpoints will download a (text) file corresponding to each endpoint. These files aren’t that useful in themselves, though, so let’s keep going.
Cloning the repo
Next, you can try cloning the repository if you find a *.git
endpoint in the previously retrieved logs.
Instead, you can use gitdumper.sh to dump the repository and make a local copy for yourself.
First, grab the gitdumper.sh script, then run:
./gitdumper.sh https://sitename.com/.git/ <local directory name>
If all goes to plan, you will now have a new directory with the name you specified, full of files for you to investigate.
Poke around
Now that you’ve got a copy, check out the previous commit messages with:
git log
Here’s an example using the git-all-secrets repo shared later in this post:
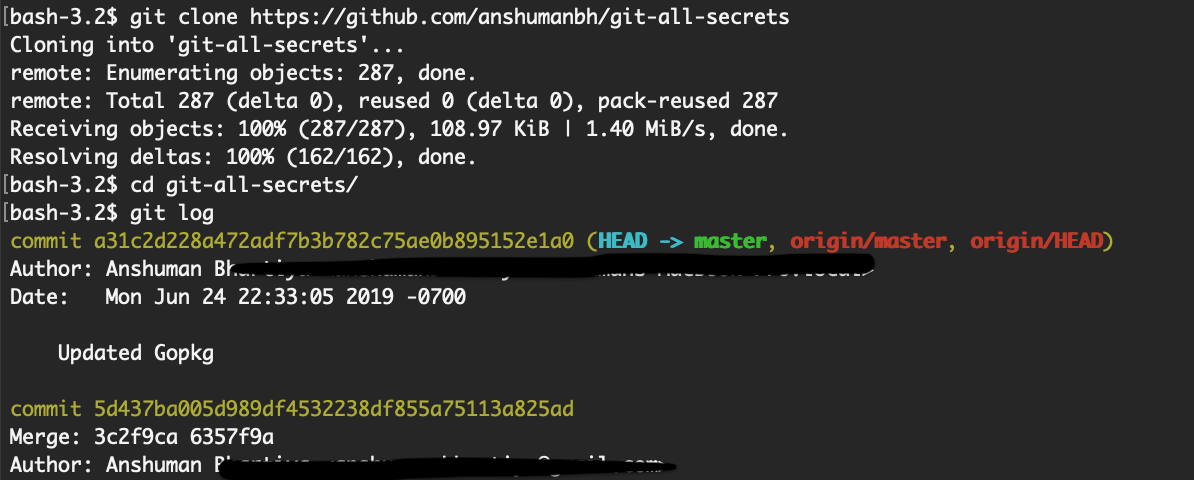
If you see a commit that looks particularly interesting (maybe one right before a “whoops removing [secret info!]” commit), find the commit hash number and do:
git checkout <commit number>
You can also view other branches with:
git branch -a
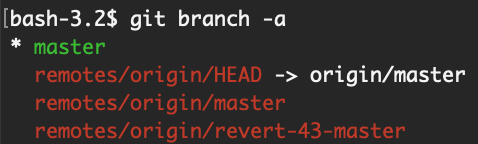
And use git checkout -b <branch name>
Lastly, try out git diff
and git status
if you’re doing a CTF challenge, as uncommitted information might have a flag (this trick seems less useful in “real life”, though).
Finding secrets in GitHub
Git itself is an open-source version control system, and while you can host your own git server, most large companies (or even individual developers) opt not to.
This is where sites like GitHub and Gitlab come in: they are hosting platforms that offer the functionality of a git server, plus some extra features (such as issue tracking, commenting, etc) and more reliability than your a personal git server might provide.
You can have private or public repositories, and while it’s widely understood that you shouldn’t upload secrets or keys in public places, mistakes do happen. Especially since there are thousands of companies and millions of developers pushing code to GitHub every day.
If you are doing security assessments or bug bounty, you might be able to find GitHub repositories owned by or related to the site or service you are testing.
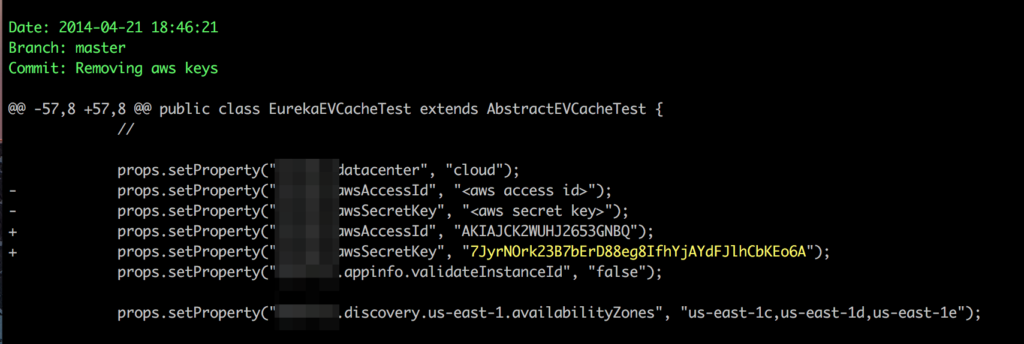
If so, you can use one of these libraries to search through those repositories:
- gitleaks: “Gitleaks is a SAST tool for detecting hardcoded secrets like passwords, api keys, and tokens in git repos. Gitleaks is an easy-to-use, all-in-one solution for finding secrets, past or present, in your code.”
- truffleHog: “Searches through git repositories for secrets, digging deep into commit history and branches. This is effective at finding secrets accidentally committed.”
- git-all-secrets: “A tool to capture all the git secrets by leveraging multiple open source git searching tools”
You can also use the repository access to understand the software better and find other vulnerabilities.
Try it out
As you find security tools on GitHub that you’d like to use, try doing a git clone
instead of downloading the project as a zip folder.
If you want to give this a try as a developer (either to commit/share your personal projects, or just for fun), I recommend signing up for a free account at GitHub, and creating a new repository.
You can also contribute to open source (which will not only require basic git skills but give you practice in merging, pull requests, and GitHub/GitLab issue tracking as well). While it’s not security-specific, FirstTimers Only has a list of beginner-friendly open source projects to contribute to.
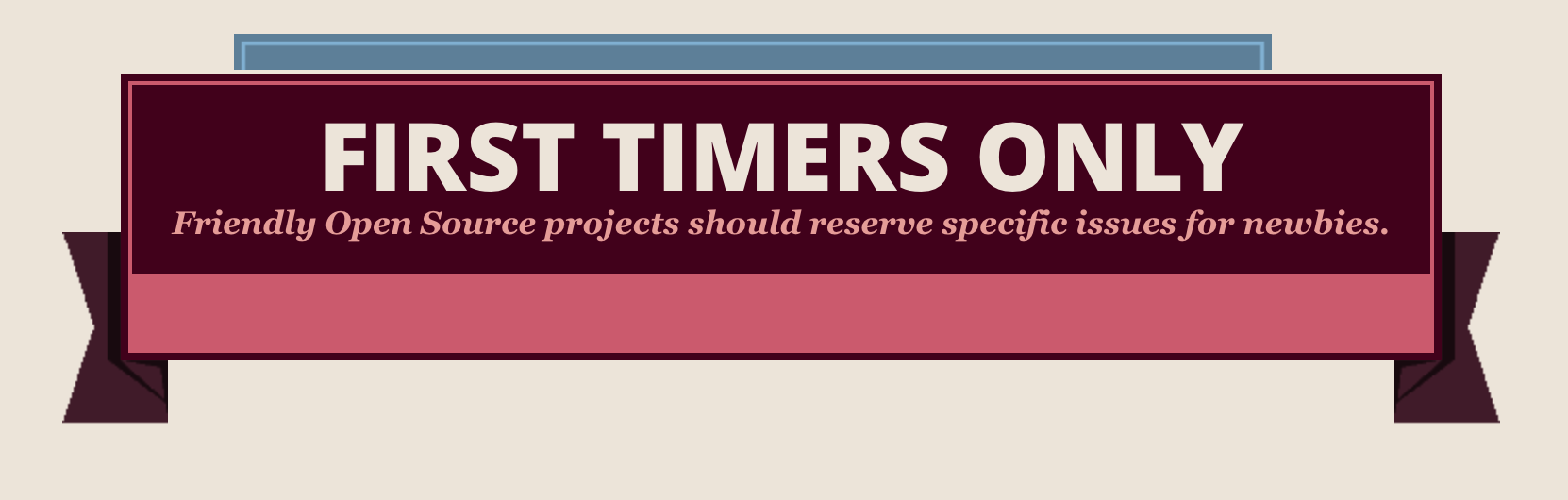
On the CTF side of things, here are two CTF writeups (1, 2) that walk through beginner git challenges.
And finally, here is a git CTF that teaches you git commands as you find flags.